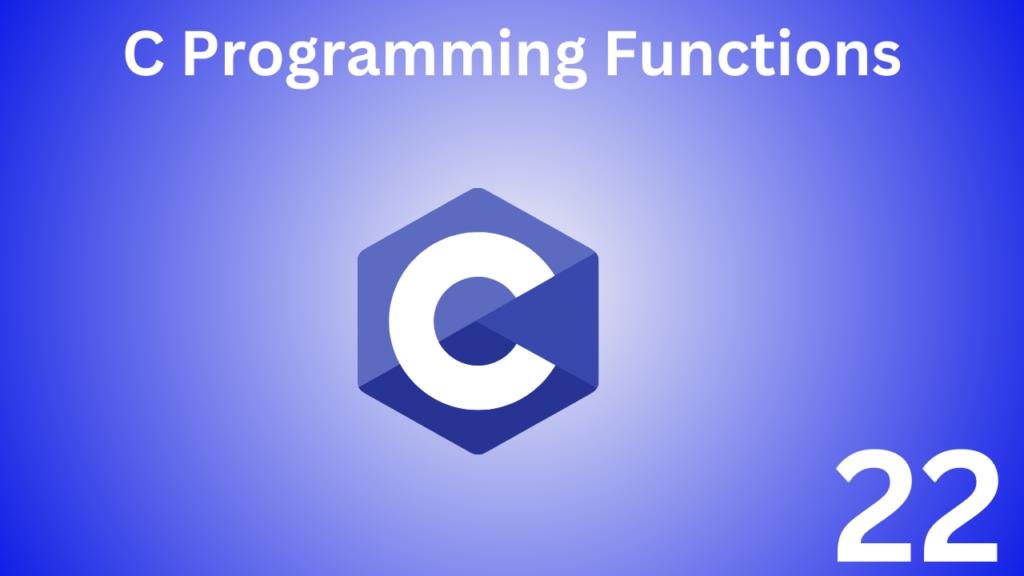
In this chapter you will be introduced to both user defined and standard library functions in C programming. You will also learn how to use programming functions.
C Programming Function
A function is a block of code that performs a specific task.
Suppose a graphics program needs a square, a circle, and some color to be specified by the user for length, radius, and color, respectively. There are three functions you can use to solve this problem.
(i) Squere Function
(ii) Circle Function
(iii) Color Function
Breaking down big problems into smaller parts makes the program easier to understand and develop.
Types of Functions in C Program
There are two types of functions in C programming based on user defined functions and functions already existing in the compiler. Namely:
(i) C standard library function
(ii) C user Defined Functions
C Standard Library Functions
Standard library functions in C programming are predefined functions of C’s own functions that can perform predefined functions that can perform mathematical calculations, input-output processing, string handling, etc.
These functions are defined in the header file. When those header file are include in a program, these functions act as their own functions in the source code. For example:
printf() is a standard library function that sends formatted output to the screen. This function “stdio.h” is defined in the header file.
Besides, there are many more functions which “stdio.h” are defined in the header file like scanf(), getchar(), fprintf() etc. “stdio.h” These function become common to your program whenever you include the header file in your program.
C User Defined Function
As already mentioned, C programming allows for function definitions. Since these functions are defined by the user, they are called user defined functions.
You can define as many functions as you want based on the requirements and complexity of the program.
How do user defined functions work?
#include
void userDefinedFunction()
{
... .. ...
... .. ...
}
int main()
{
... .. ...
... .. ...
userDefinedFunction();
... .. ...
... .. ...
}
C program execution starts main() from function.
When the compiler userDefinedFunction(); encounters a function, program control jumps void userDefinedFuntion() to it.
The compiler then starts executing the code in the user defined function.
When user-defined function codes complete execution, program control jumps back to main() the function itself. userDefinedFunction();
Remember, function names are identifiers that must be unique.
This is an overview of user defined functions. Visit the following page to know more:
(i) C User Defined Function
(ii) Types of User Defined Functions
Benefits of User Defined Functions
- Program understanding, maintenance and debugging become easier.
- Code can also be used in other programs due to increased reusability,
- Larger programs can be broken down down into smaller modules. So large projects can be divided among many programmers.
Pingback: String Manipulation Using Library Functions -
Pingback: How to pass structure through function in C programming? - Quick Learn
Pingback: C Call by Reference: Using pointers - Quick Learn