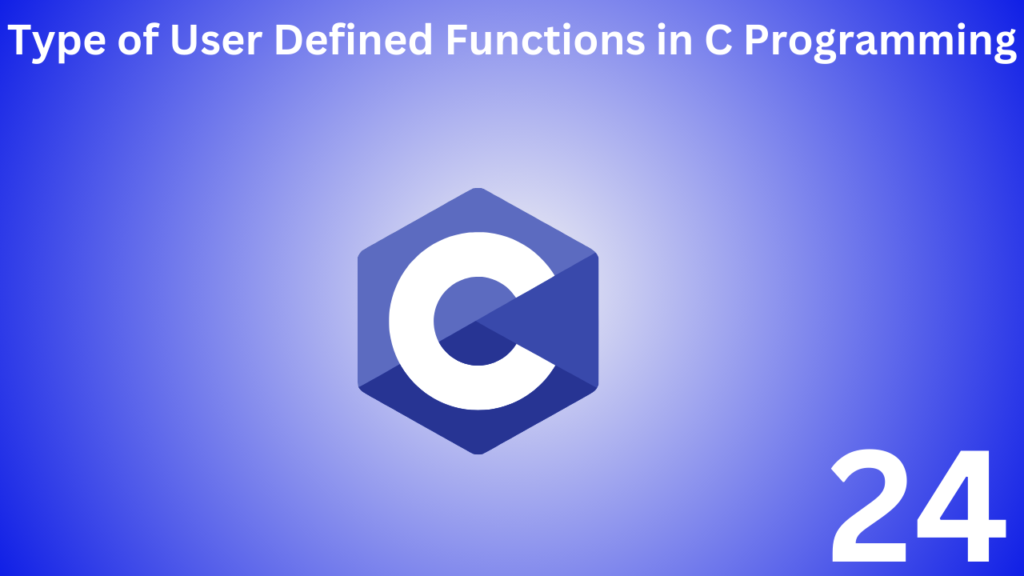
In this chapter you will learn to solve the same problem in different ways using functions.
Table of Contents
C User Defined Function
To better understand the functions return values and arguments, user defined functions can be divided into the following categories:
- Functions with arguments and return values
- Function without arguments and return values
- Function with out arguments and with return values
- Function with arguments and without return value
Below is a program to check if the user enters a prime number in each of the following four programs. All programs show the same result.
Example: Functions with arguments and return values
#include
int checkPrimeNumber(int n);
int main()
{
int n, flags;
printf("Enter a positive integer: ");
scanf("%d",&n);
/* n is passed through the checkPrimeNumber() function.
The value returned from the function is stored in the flag variable*/
flag = checkPrimeNumber(n);
if(flag==1)
printf("%d is not a prime number",n);
else
printf("%d is a prime number",n);
return 0;
}
// This function returns an integer.
int checkPrimeNumber(int n)
{
/*Integer number is returned from checkPrimeNumber() function */
int i;
for(i=2; i <= n/2; ++i)
{
if(n%i == 0)
return 1;
}
return 0;
}
Input by the user checkPrimeNumber() is passed through the function.
checkPrimeNumber() The function checks whether the argument passed is a prime number. The function returns 0 if the passe argument is a prime number. Return 1 if the passed argument is not prime. The return value is assigned to the flag variable.
Finally main() the appropriate message from the function is displayed.
Example: Functions without arguments and return value
#include
void checkPrimeNumber();
int main()
{
checkPrimeNumber(); // No arguments were passed to the checkPrimeNumber() function.
return 0;
}
// Since no value is returned from the function, the return type is kept void.
void checkPrimeNumber()
{
int n, i, flag=0;
printf("Enter a positive integer: ");
scanf("%d",&n);
for(i=2; i <= n/2; ++i)
{
if(n%i == 0)
{
flag = 1;
}
}
if (flag == 1)
printf("%d is not a prime number.", n);
else
printf("%d is a prime number.", n);
}
checkPrimeNumber() The function accepts input from the user, checks whether the input value is a prime number, and displays an appropriate message on the screen.
main() The empty parentheses of the function within the function checkPrimeNumber(); indicate that no values can be passed through the function.
checkPrimeNumber(); As the return type of the function, void it does not return any value.
Example: Functions without arguments and with return values
#include
int getInteger();
int main()
{
int n, i, flag = 0;
//No arguments passed to the function.
// The value returned from the function is stored in the n variable.
n = getInteger();
for(i=2; i<=n/2; ++i)
{
if(n%i==0){
flag = 1;
break;
}
}
if (flag == 1)
printf("%d is not a prime number.", n);
else
printf("%d is a prime number.", n);
return 0;
}
// Returns the Integer number from the getInteger() function.
int getInteger()
{
int n;
printf("Enter a positive integer: ");
scanf("%d",&n);
return n;
}
n = getInteger(); The empty parentheses of the function indicate which value will be passed through the function and the return value from the function will be assigned to the n variable.
Here getInteger() the function takes input from the user and returns it. The code to check if the number is prime main() is in the function.
Example: Function with arguments and without return value
#include
void checkPrimeAndDisplay(int n);
int main()
{
int n;
printf("Enter a positive integer: ");
scanf("%d",&n);
// n is passed to the function
checkPrimeAndDisplay(n);
return 0;
}
// void indicates that no value is returned from the function
void checkPrimeAndDisplay(int n)
{
int i, flag = 0;
for(i=2; i <= n/2; ++i)
{
if(n%i == 0){
flag = 1;
break;
}
}
if(flag == 1)
printf("%d is not a prime number.",n);
else
printf("%d is a prime number.", n);
}
The integer number entered by the user checkPrimeAndDisplay() is passed through the function.
checkPrimeAndDisplay() The function checks whether the argument passed is a prime number and displays the appropiate message.
Which method is best?
It depends on what kind of problem you want to solve. The first one is best for our problem.
Function should be used to achieve specific tasks. In our first program checkPrimeNumber() the function does not accept any input from the use and does not display any messages. It only checks if the number is prime which makes the program modular. This makes the program easier to understand and debug.