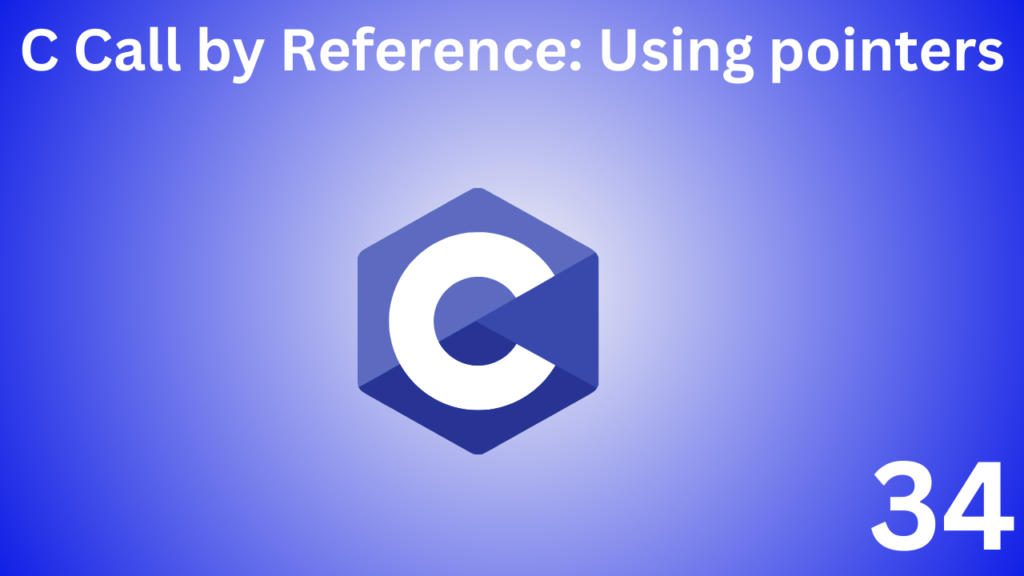
C Call by Reference: Using pointers
In this chapter you will learn how to pass pointers as arguments to functions. Also learn how to use pointers correctly in your program.
Pointer: Function call by reference
When a pointer is passed as an argument to a function , the address of the memory location is passed instead of the value.
Because in C programming, instead of storing the pointer value, the memory location is stored as the value.
Example: Use of pointers and functions
C program to swap two numbers by calling a function by reference
/* C program to swap two numbers using pointers and functions*/
#include
void swap(int *n1, int *n2);
int main()
{
int num1 = 5, num2 = 10;
// The addresses of num1 and num2 are passed through the swap() function.
swap( &num1, &num2);
printf("Number1 = %d\n", num1);
printf("Number2 = %d", num2);
return 0;
}
void swap(int * n1, int * n2)
{
// The pointers n1 and n2 point to the addresses of num1 and num2 respectively.
int temp;
temp = *n1;
*n1 = *n2;
*n2 = temp;
}
output
Number1 = 10
Number2 = 5
Explanation of the above example:
- The memory location addresses of num1 and num2 variables are passed to the swap() function. Pointers *n1 and *n2 take those addresses as values.
- So now pointer n1 and n2 point to address of num1 and num2 respectively .
- When the value of the pointer changes, the value pointed to in the memory location also changes accordingly.
- So a change in pointer *n1and also changes in function num1 and num2 .*n2 main()
This technique is called call by reference in C programming.