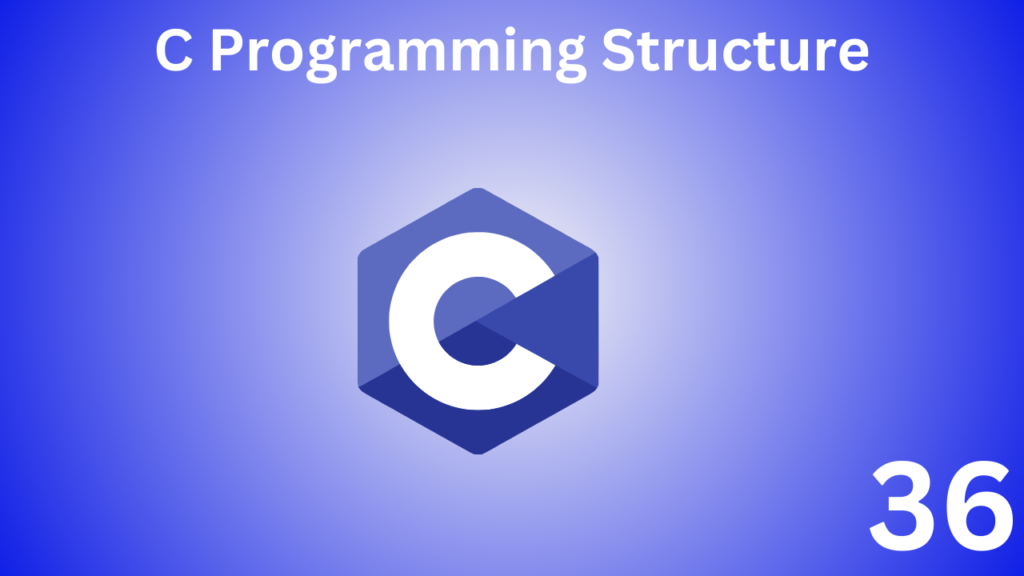
C programming structure
Structures in C programming are user defined data types.
Structures are collections of variables with different data types under a single name.
For example: You want to collect information about a person (name, ID and address). You can easily create various variables to store individual information. Like name, idNo, address etc.
However, if you want to store the data of many persons then you need to create different variables for each person like name1, idNo1, address1, name2, idNo2, address2 etc.
As you can probably imagine, the code will be very long and look messy. Also, since there is no relationship between the variables (data), it is going to become a scary task.
Person
A simpler and more accurate method would be to group the related information into a single name and use it for each person. Now the code will look much more transparent and readable and the efficiency of the code will also increase.
Person
A structure is a collection of information related by a single name .
C structure definition
struct
Structure is created through keywords.
Syntax of structures
struct structure_name
{
data_type member1;
data_type member2;
.
.
data_type memeber;
};
Note: Do not put semicolon(;) at the end of the line.
For the above person
we can create the following structure:
struct person
{
char name[50];
int idNo;
float address;
};
The above declaration struct person
creates the derived data type.
Structure variable declaration
When a structure is defined, it creates a user-defined data type. But no memory or storage is allocated.
For the above person structure, variables can be declared as follows:
struct person
{
char name[50];
int idNo;
float address;
};
int main()
{
struct person person1, person2, person3[30];
return 0;
}
Another method of declaring a structure variable:
struct person
{
char name[30];
int idNo;
float address;
} person1, person2, person3[30];
In both cases person1 and person2 are variables and the data type of person3 array is person
Accessing the members of a structure
There are two types of operators to access the members of a structure.
1. Member operator(.)
2. Structure pointer operator(->)
The member variables of the structure can be accessed as follows:
structure_variable_name.member_name
Suppose we want to access the address of variable person1 then it can be accessed as below.
person1.address
Examples of structures
C program to add two distances with value input by user. Use inches and feet to measure distances. (Note: 12 inches = 1 foot)
#include
struct Distance
{
int feet;
float inch;
} dist1, dist2, sum;
int main()
{
printf("1st distance\n");
// Input feet value for structure variable dist1.
printf("Enter feet: ");
scanf("%d", &dist1.feet);
// Input inches value for structure variable dist1.
printf("Enter inch: ");
scanf("%f", &dist1.inch);
printf("2nd distance\n");
// Input feet value for structure variable dist2.
printf("Enter feet: ");
scanf("%d", &dist2.feet);
// Input inches value for structure variable dist2.
printf("Enter inch: ");
scanf("%f", &dist2.inch);
sum.feet = dist1.feet + dist2.feet;
sum.inch = dist1.inch + dist2.inch;
if (sum.inch > 12)
{
//If the inch value is greater than 12, it will be converted to feet.
++sum.feet;
sum.inch = sum.inch - 12;
}
//Print the sum of distances dist1 and dist2
printf("Sum of distances = %d\'-%.1f\"", sum.feet, sum.inch);
return 0;
}
output
1st distance
Enter feet: 12
Enter inch: 7.9
2nd distance
Enter feet: 2
Enter inch: 9.8
Sum of distances = 15'-5.7"
Requirement of typedef keyword when using structure
struct structure_name variable_name;
Using structure to declare variables takes more time for development. As a result, struct
the significance of the use has to be deprived.
typedef
So developers usually use keywords to name structures . For example:
typedef struct complex
{
int imag;
float real;
} comp;
int main()
{
comp comp1, comp2;
}
Here the comp type is created typedef
using the keyword where the type of com is ).struct complex
Then two structure variables comp1 and comp2 of type comp are created.
Structure within structure
In C programming, a structure can be nested within another structure.
struct complex
{
int imaginary_value;
float real_value;
};
struct number
{
struct complex comp;
int real;
} number1, number2;
Suppose you want to access the imaginary_value of the number2 structure variable , then the following structure member will be used:
number2.comp.imaginary_value
Passing structures through functions
There are two methods of passing structures through functions:
- Passing by value
- Passing by reference
This is discussed in detail in the Structure and Function chapter.
Pingback: C Programming Structure and Pointer - Quick Learn
Pingback: How to pass structure through function in C programming? - Quick Learn
Pingback: C Programming Bit Field - Quick Learn