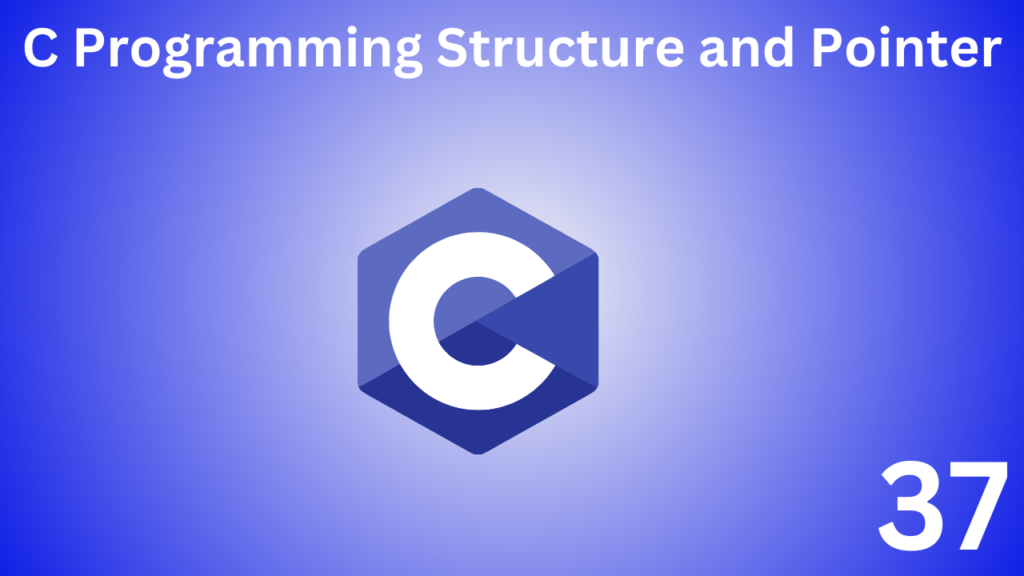
Table of Contents
ToggleC Programming Structures and Pointers
Structures can be both created and accessed using pointers . A pointer variable of structure type can be created as follows:
struct name {
member1;
member2;
.
.
};
int main()
{
struct name *ptr;
}
struct name
A pointer variable of type(*ptr) is created in the above program .
Accessing structure members through pointers
Structure members can be accessed through pointers in two ways:
- Accessing memory by referring a pointer to another address
- Using dynamic memory allocation.
1. Accessing memory by referring a pointer to another address
C program to access structure member through pointer:
#include
typedef struct person
{
int age;
float weight;
};
int main()
{
struct person *personPtr, person1;
personPtr = &person1; //Refer pointer to memory address of person1
printf("Enter integer: ");
scanf("%d",&(*personPtr).age);
printf("Enter number: ");
scanf("%f",&(*personPtr).weight);
printf("Displaying: ");
printf("%d%f",(*personPtr).age,(*personPtr).weight);
return 0;
}
In the example above, struct person
the type pointer variable is referred to the address of the person1 variable. So structure members can be accessed only through pointers.
->(Arrow) operator is used to access the pointer member of the structure.
.(Dot)
You can also access pointer members of structures using operators.
(*personPtr).age and personPtr->age are the same
(*personPtr).weight and personPtr->weight are the same
2. Accessing structure members through pointers using dynamic memory allocation
Memory can be dynamically allocated using the malloc() function to access structure members through pointers .
Syntax of using malloc()
ptr = (cast-type*) malloc(byte-size)
C program to access structure members through pointers using malloc() function
#include
#include
struct person {
int age;
float weight;
char name[30];
};
int main()
{
struct person *personPtr;
int i,num;
printf("Enter number of persons: ");
scanf("%d", &num);
personPtr = (struct person*) malloc(n * sizeof(struct person));
//The above statement allocates memory for n number of structures for the personPtr pointer */
for(i = 0; i < n; ++i)
{
printf("Enter name, age and weight of the person respectively:\n");
scanf("%s%d%f", &(ptr+i)->name, &(ptr+i)->age, &(ptr+i)->weight);
}
printf("Displaying Infromation:\n");
for(i = 0; i < num; ++i)
printf("%s\t%d\t%.2f\n", (ptr+i)->name, (ptr+i)->age, (ptr+i)->weight);
return 0;
}
output
Enter number of persons: 2
Enter name, age and weight of the person respectively:
Adam
2
3.2
Enter name, age and weight of the person respectively:
Eve
6
2.3
Displaying Information
Adam 2 3.20
Eve 6 2.30