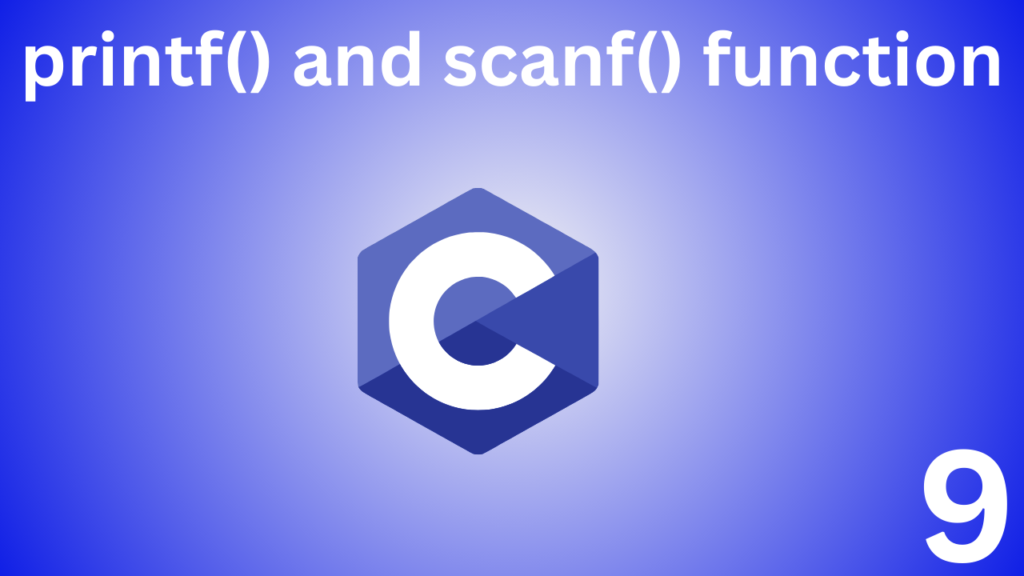
Table of Contents
C print() and scanf() Function
In this chapter we will focus more on printf() and scanf(), two built-in functions of C programming that are used to perform input and output operations. Besides, in this chapter you will also learn to write valid C programs.
C Programming Input-Output (I/O)
C programming has some built-in library functions to perform input and output functions.
The two most commonly used functions for input-output (I/O) in C programming are printf() and scanf().
scanf() The functions is used to take formatted input from the user through the standard input device (keyboard). On the other hand printf(), the function is used to send formatted output to the standard output (screen).
C Programming input syntax
scanf("%c", &var_name); //Character input
scanf("%d", &var_name); //integer input
scanf("%f", &var_name); //float input
scanf("%lf", &var_name); //double input
scanf("%s", &var_name); //string input
C Programming Output Syntax
printf("%c", &var_name); //Character output
printf("%d", &var_name); //integer output
printf("%f", &var_name); //float output
printf("%lf", &var_name); //double output
printf("%s", &var_name); //string output
Example: C Output
C Programming
#include
int main()
{
printf("C Programming");
return 0;
}
In this chapter we will focus more on printf() and scanf(), two built-in functions of C programming that are used to perform input and output operations. Besides, in this chapter you will also learn to write valid C programs.
- All C programs must main() have functions. main() Code execution starts from the beginning of the function.
- printf() is a library functions that sends formatted output to the screen. Functions are declared "stdio.h" in the header file. printf()
- Here stdio.h is the standard input-output header file and #include the preprocessor that links the header file to the source code. When the compiler printf() executes the function and stdio.h can't find the header file, the compiler throws and error.
- return 0; statement exits the program. Teminates the program in plain language.
Example: C Character input/output
#include
int main()
{
char character;
printf("Enter a character: ");
scanf("%c",&character); // The %c format string is used for characters
printf("You entered %c.",character);
return 0;
}
Enter a character: t
You entered t.
The “%c” format string is used to accept formatted character input and send output.
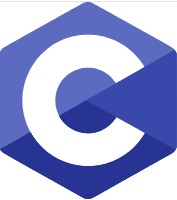
Example: C Integer Output
#include
int main()
{
int integerNumber = 5; //The %d format string is used for integers
printf("Number = %d", integerNumber);
return 0;
}
Number = 5
printf() “%d” The function contains the fomatted string for integers within quotation marks. In this case, if the format string matches the integerNumber argument, it displays the output on the screen.
Example: C integer input/output
Enter an integer number: 4
Number = 4
scanf() The function accepts formatted input from the keyboard. When the user inputs an integer number, it is stored in the IntegerNumber variable.
Notice that IntegerNumber ‘&‘ has a sign before it. &IntegerNumber points to the address or IntegerNumber and the input value is stored at that address.
Example: C float input/output
#include
int main()
{
float floatNumber;
printf("Enter a number: ");
//%f format string is used for floats
scanf("%f",&floatNumber);
printf("Value = %f", floatNumber);
return 0;
}
Enter a number: 23.45
value = 23.450000
The “%f” format string is used to receive formatted input and send output for floats.
ASCII (ASCII) Code
When a character is entered in the above program, the character is not saved in its own format. Insted, numbers are stored in value (ascii value) format.
When we “%c” print using text format it shows on screen in character format.
Example: ASCII Code
#include
int main()
{
char character;
printf("Enter a character: ");
scanf("%c",&character);
//When we print using "%c" text format it shows on screen in character format.
printf("You entered %c.\n",character);
//When we print using "%d" text format it shows on screen in integer format.
return 0;
}
Enter a character: b
You entered b.
ASCII value of g is 96.
ASCII value of character “b” is 96. When ‘b’ is entered 96 is stored in the character variable instead of ‘b’.
If you know the ASCII code of a character, you can output it as a character. This is shown in the folloing example:
Example: ASCII Code
#include
int main()
{
int character = 70;
printf("Character of ASCII value 70 is %c.",character);
return 0;
}
Character of ASCII value 70 is F.
Pingback: C Programming Operators -