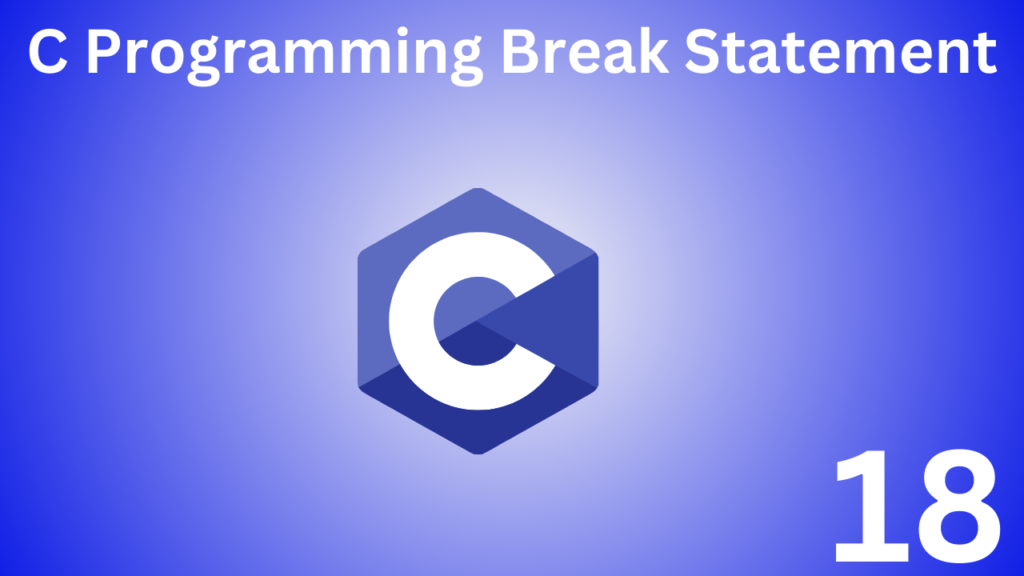
C Programming Break Statement
Table of Contents
break Statements are used in programming to terninate the normal flow of loops. In this chapter, you break will learn how to immediately terminate various loops in c programming using statements.
Sometimes it is necessary to terminate the loop immediately without checking the test expression. In this case break statement is used.
C Programming break statement
The break statements for, while and do…while loops terminate immediately as soon as the c program break statement is encountered. The break statement is used in decision-making statements such as if…else statements.
Syntax of break statement
break;
Example 1: Break Statement
#include
#include
int main()
{
int i=1;//initialized local variable.
clrscr();
//will start the loop from 1 to 10
for(i=1;i<=10;i++)
{
printf("%d \n",i);
if(i==7){
// The loop will stop if the value of i is equal to 7
break;
}
}// End of for loop
getch();
}
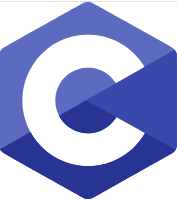
output
1
2
3
4
5
6
7
Example 2: Break Statement
// Program to add up to 15 numbers
//The sum continues until the user enters a positive number.
# include
int main()
{
int i;
double number, sum = 0.0;
for(i=1; i <= 15; ++i)
{
printf("Enter a number%d: ",i);
scanf("%lf",&number);
// The loop ends as soon as the user enters a negative number.
if(number < 0.0)
{
break;
}
sum += number; // sum = sum + number;
}
printf("Sum = %.2lf",sum);
return 0;
}
output
Enter a number1: 5
Enter a number2: 5.7
Enter a number3: 10
Enter a number4: 8.4
Enter a number5: 2.5
Enter a number6: -5
Sum = 31.5
The above program calculates the sum of up to 15 numbers. But for loop terminates immediately when the break statement is encountered at input number 6.
Break statement is also used in switch…case statement in C programming.