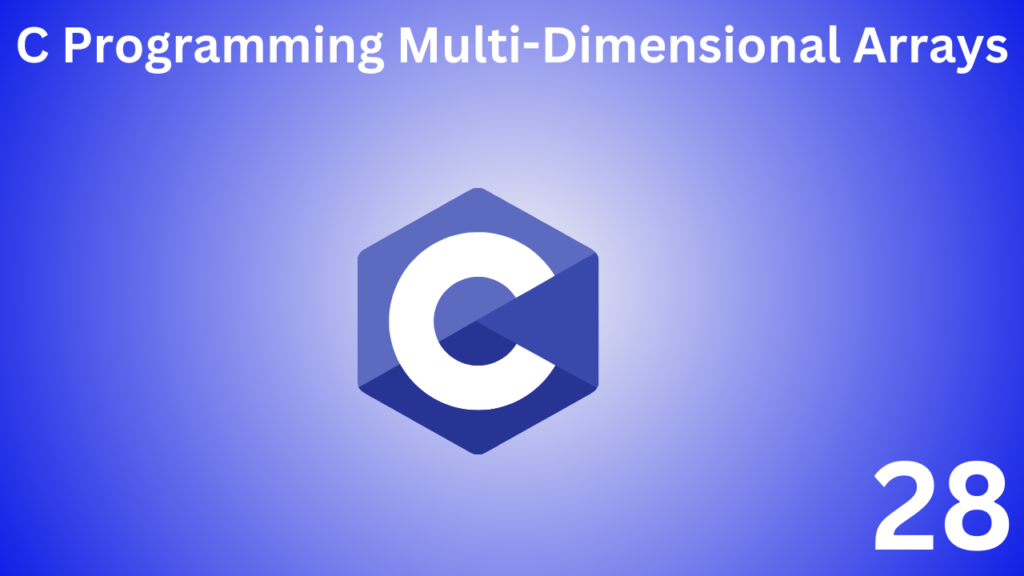
C programming offers a powerful feature known as multi-dimensional arrays, which allows you to store data in a structured way. Whether you’re dealing with matrices, grids, or even more complex data structures, understanding how to effectively use multi-dimensional arrays can significantly enhance your coding skills. In this blog post, we’ll dive into what multi-dimensional arrays are, how to declare and initialize them, and some practical examples to illustrate their usage.
What Are Multi-Dimensional Arrays?
A multi-dimensional array is essentially an array of arrays. While a one-dimensional array holds a list of items (like integers or characters), a two-dimensional array can be visualized as a table with rows and columns. In C, you can create arrays with three or more dimensions as well, but two dimensions are the most common.
Syntax
The syntax for declaring a multi-dimensional array is:
data_type array_name[size1][size2]...[sizeN];
For example, to declare a two-dimensional array of integers with 3 rows and 4 columns:
int matrix[3][4];
Initializing Multi-Dimensional Arrays
You can initialize a multi-dimensional array at the time of declaration. Here’s how to do it:
int matrix[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
In this example, the matrix
contains three rows and four columns. Each set of braces represents a row in the array.
Accessing Elements
Accessing elements in a multi-dimensional array is similar to accessing elements in a one-dimensional array, with the addition of another index. The syntax is:
array_name[row][column]
For example, to access the element in the second row and third column of the matrix
:
int value = matrix[1][2]; // value will be 7
Example: Basic Operations on a 2D Array
Let’s look at a complete example that demonstrates basic operations like initializing, displaying, and modifying a 2D array.
#include
int main() {
// Initialize a 3x4 matrix
int matrix[3][4] = {
{1, 2, 3, 4},
{5, 6, 7, 8},
{9, 10, 11, 12}
};
// Display the matrix
printf("Original Matrix:\n");
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
printf("%d ", matrix[i][j]);
}
printf("\n");
}
// Modify an element
matrix[1][2] = 99; // Changing 7 to 99
// Display the modified matrix
printf("\nModified Matrix:\n");
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 4; j++) {
printf("%d ", matrix[i][j]);
}
printf("\n");
}
return 0;
}
Output
Original Matrix:
1 2 3 4
5 6 7 8
9 10 11 12
Modified Matrix:
1 2 3 4
5 6 99 8
9 10 11 12
Applications of Multi-Dimensional Arrays
Multi-dimensional arrays are widely used in various applications, including:
- Matrices in Mathematics: Performing operations like addition, subtraction, and multiplication.
- Game Development: Representing game boards, grids, or maps.
- Image Processing: Storing pixel values in two-dimensional arrays.
- Scientific Computations: Handling data sets that require multiple dimensions.
Conclusion
Multi-dimensional arrays are a fundamental concept in C programming that provide a way to store and manipulate data efficiently. They open up new possibilities for handling complex data structures and can significantly simplify your code. By mastering multi-dimensional arrays, you can improve your programming skills and tackle more challenging projects with confidence.