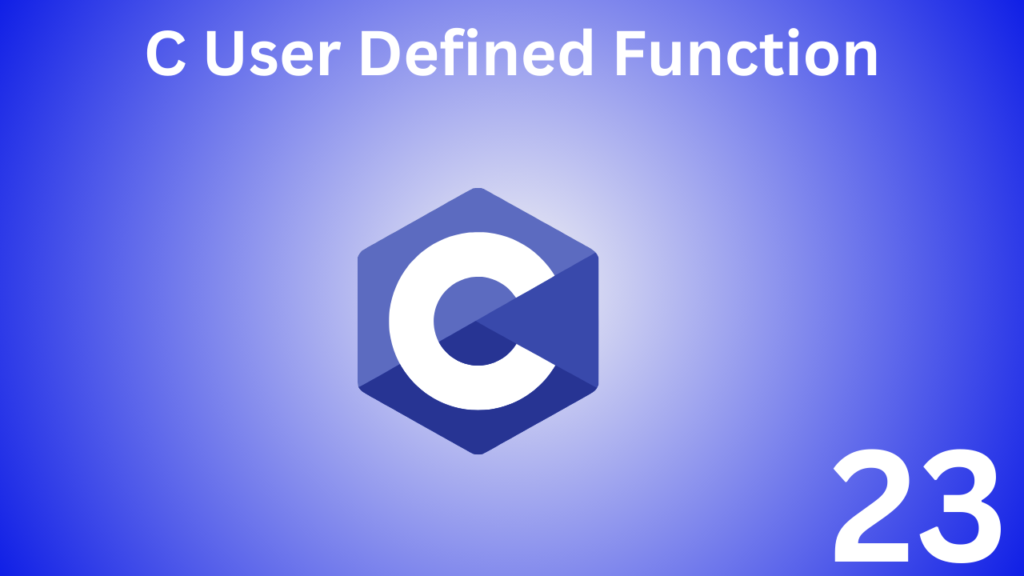
C programming allows defining functions. In this chapter you will learn how to create user defined functions in C programming.
Table of Contents
C User Defined Functions
A function is a block of code that performs a specific task.
C programming allows you to define functions as per your needs. These functions are known as user defined functions. For example:
Suppose a graphics program needs a square, a circle, and a color that depends on the lenghth, radius, and color specified by the user, respectively. There are three functions you can use to solve this problem.
(i) square function
(ii) circle function
(iii) color function
Example: C User Defined Functions
The following example shows the program for multiplying two integer numbers. A user defined function multiplyNumbers() is defined to perform this task.
#include
int multiplyNumbers(int a, int b); // function prototype
int main()
{
int num1,num2,multiplication;
printf("Enter two numbers: ");
scanf("%d %d",&num1,&num2);
multiplication = multiplyNumbers(num1, num2); //function call
printf("multiplication = %d",multiplication);
return 0;
}
int multiplyNumbers(int a,int b) //function definition
{
int result;
result = a*b;
return result; // return statement
}
C Function Prototype
A function prototype usually refers to a function declaration that defines the function name, parameters, and return type. It does not contain the body of the body of the function i.e. the function definition.
The function prototype gives the compiler advance notice that the function may be used later in the program.
Syntax of C function prototype
returnType functionName(type1 argument1, type2 argument2,...);
The above program int multiplyNumbers(int a, int b); contains function prototypes that provide the following information to the compiler:
- multiplyNumbers() is the name of the function.
- int is the return type of the function
- int two arguments of type will be passed through the function.
A funciton prototype is not required if the user defined function main() is previously defined.
Calling a C function
By calling the program control is transferred to the user defined function.
Syntax of C function calls
functionName(argument1, argument2, ...);
In the example above, the function is called using the main() within-function statement. multiplyNumbers(n1,n2);
Syntax of C function calls
A function definition consists of a block of code to perform a specific task. For example, the above example uses some code to multiply two numbers between the functions return type and the function definition.
Syntax of C function definitions
returnType functionName(type1 argument1, type2 argument2, ...)
{
//body of the function
}
Control of the program is transferred to the function definition when the function is called. The compiler starts executing the code inside the function body.
Passing arguments to functions
In programming, arguments refer to variable that are passed through functions. In the above example the num1 and num2 variables are passed between the two functions during the function call.
The function definition takes two arguments, passing parameners a and b. These arguments are called formal parameters of the function.
The arguments and formal parameters passed to the function must be the same or the compiler will throw an error.
If num1 is of type Integer then a must be of type Integer. If num2 is of type double then variable b must be of type double.
Function can be called without passing arguments to the function.
Syntax of C return statements
return (expression);
For example
return sum;
return (x+y);
The type of the value rerurned from the function and the return type of the function prototype and function definition must be the same.