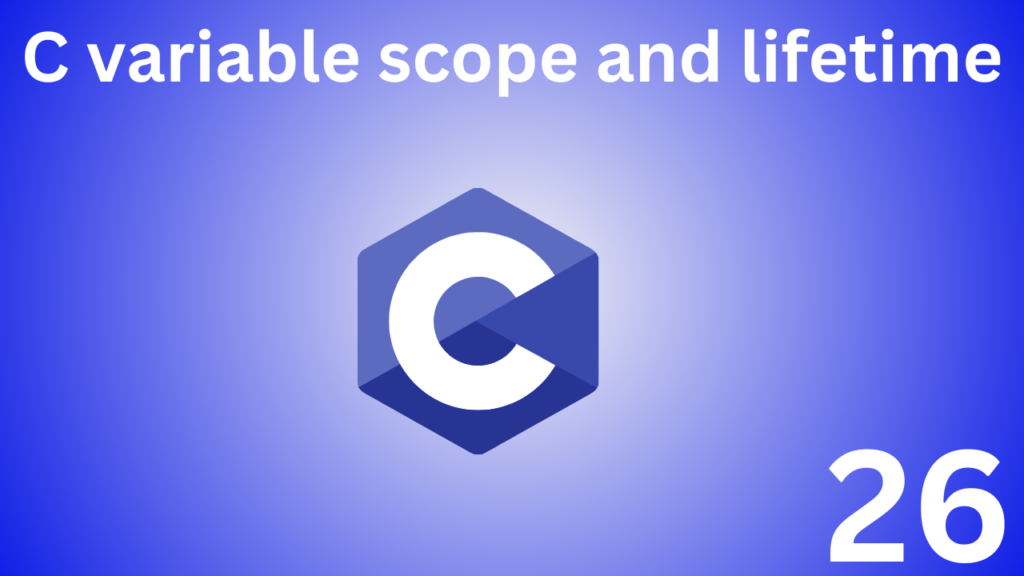
In this chapter you will learn about the scope and lifetime of local and global variables. Aslo learn about static and register variables.
Table of Contents
Scope and lifetime of C Varible
In C programming every variable has two attributes. Namely:
(i) Type
(ii) Storage Class
Type refers to the data type of the variable and the storage class determines the scope and lifetime of the variable.
There are four types of storage classess in C programming.
(i) Automatic
(ii) External-external
(iii) Static-static
(iv) Register-register
C is local variable
Variable declared within a function are called automatic of local variables.
Local variables exist only within functions. The local variable is destroyed when the function is done.
int main() {
int num; // Here num is local variable of main() function
... .. ...
}
void add() {
int num1; // Here num1 is local variable of add() function
... .. ...
}
C Global Variable
Variable declared outside the function are called global of external variables. Global or external variables can be accessed from any function.
Example: Global Variable
#include
void display();
int count = 3; // Global variable
int main()
{
//count variable is not declared in main() function
++count;
display();
return 0;
}
void display()
{
// count variable is not declared in display() function
++count;
printf("count = %d", count);
}
count = 5
The Extern keyword
Suppose a global variable file1 is declared as if you file2 try to access that variable from another file, the compiler will complain.
To solve this problem, the file2 te extern keyword is used to indicate to the file that the external variable declared in a different file.
C register variable
In C programming, register the keyword register is used to declare variables. Register variable are considered faster than local variables.
However, modern compilers are very good at code optimization, and programs using register variables have very little chance of speeding up.
There is no need to user register variable unless you know in advance how to optimize the code for the application used on the extended system.
C static variable
static Static is used in C programming. For example:
static int age;
When the function is finished in the program, the local variables inside it are also finished. static The keyword is used to keep a local variable alive until the end of the program.
The value of a static variable exists until the program ends.
Example: Static variable
#include
void display();
int main()
{
display();
display();
}
void display()
{
static int count = 0;
printf("%d ",count);
count += 10;
}
Output
0
10
In the first function call the value of the cunt variable is0, in the second function call its value is incremented to 10.
The value of the count variable is not reassigned to 0 during the second function call. Because count is static variable here. Finally 10 is displayed on the screen.