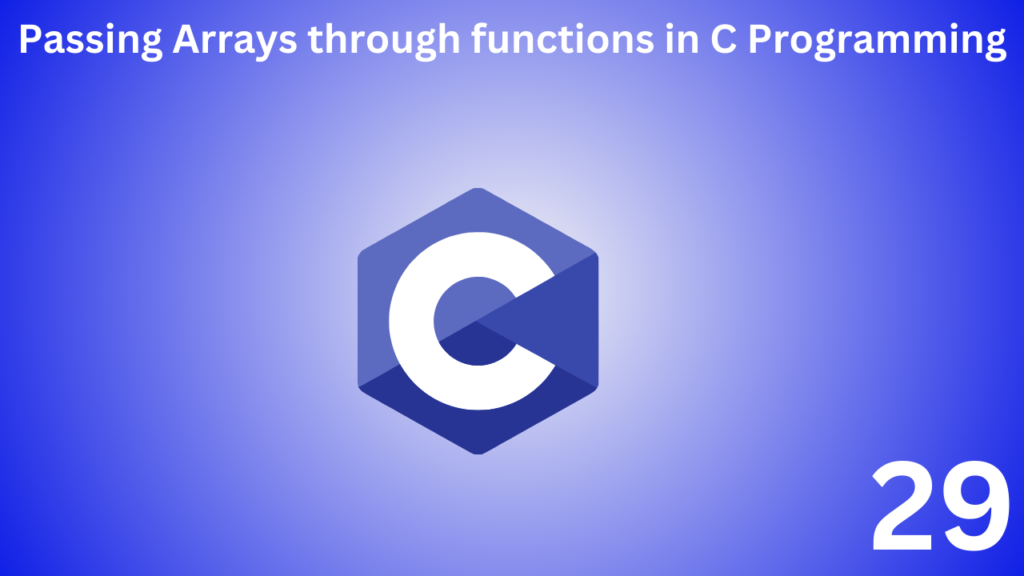
In C programming , an element of an array or the entire array can be passed through a function .
This can be done for both one-dimensional and multi-dimensional arrays.
Table of Contents
Passing One-Dimensional Arrays Through Functions
A single element of an array can also be passed in the same manner as a variable is passed through a function.
Program to traverse a single element of an array through a function
#include
void display(int age)
{
printf("%d", age);
}
int main()
{
int ageArray[] = { 2, 3, 4 };
display(ageArray[1]);
// The array element ageArray[1] is passed to the function only.
return 0;
}
3
Passing an entire one-dimensional array through a function
When an array is passed as an argument to a function, only the name of the array is passed. That is, the very beginning of the memory area is passed as an argument.
C program to pass array containing age of person through function. Through this function, the average age of the person is determined and displayed through the main function.
#include
float average(float age[]);
int main()
{
float avg, age[] = { 23.4, 55, 22.6, 3, 40.5, 18 };
avg = average(age); /* Only the name of the array is passed as an argument. */
printf("Average age=%.2f", avg);
return 0;
}
float average(float age[])
{
int i;
float avg, sum = 0.0;
for (i = 0; i < 6; ++i) {
sum += age[i];
}
avg = (sum / 6);
return avg;
}
Average age=27.08
Passing multi-dimensional arrays through functions
When passing a tow-dimensional array as an argument to a function, it traverses from the very beginning of the stored memory area, just like a one-dimensional array.
Example: Passing a two-dimensional array through a function
#include
void displayNumbers(int num[2][2]);
int main()
{
int num[2][2], i, j;
printf("Enter 4 numbers:\n");
for (i = 0; i < 2; ++i)
for (j = 0; j < 2; ++j)
scanf("%d", &num[i][j]);
// Pass the multi-dimensional array through the displayNumbers function
displayNumbers(num);
return 0;
}
void displayNumbers(int num[2][2])
{
// Except the above line void displayNumbers(int num[][2]) is also valid.
int i, j;
printf("Displaying:\n");
for (i = 0; i < 2; ++i)
for (j = 0; j < 2; ++j)
printf("%d\n", num[i][j]);
}
Enter 4 numbers:
2
3
4
5
Displaying:
2
3
4
5