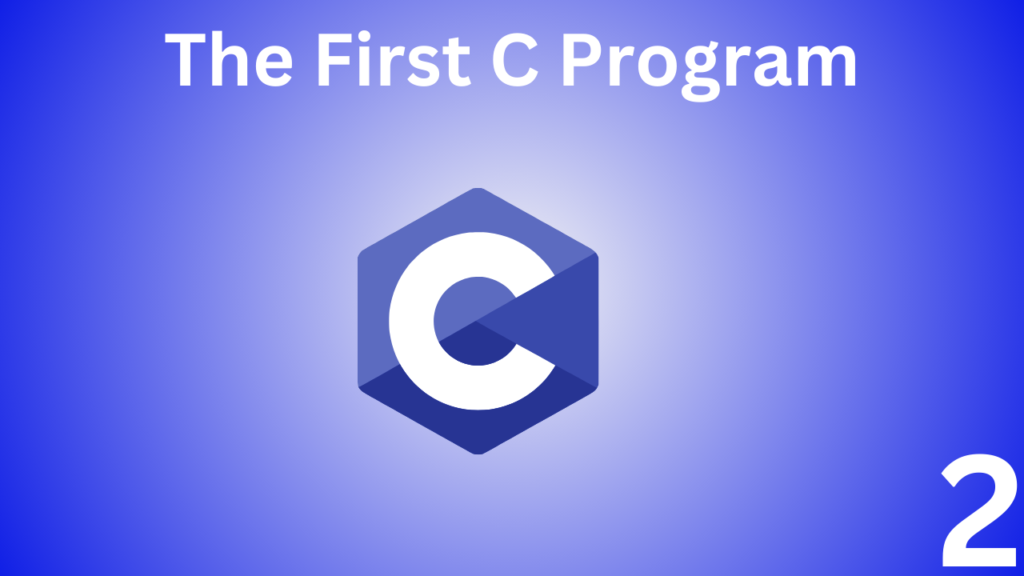
The First C Program
Table of Contents
In this chapter you will learn “Hello, World!” Also learn to write programs.
“Hello, World!” Before writing the program, let’s know what elements a typical programming language consists of.
C Programming has the following elements/parts:
- C Pre-Processor Commands
- C Comment
- C Variable
- C Function
- C Statements and Expressions
Why "Hello, World!" Program ?
“Hello, World!” is a very simple program that displays “Hello, World!” on the screen. will display It is used to describe the syntax of a Programming language as it is a very simple program.
It is used to introduce a programming language easily to beginners. So let’s begin:
#include
int main()
{
printf("Hello, World!\n");
return 0;
}
"Hello, World!" How does the Program Work ?
stdio.h Add the header file to your program.
Since C programming is short, it can’t do much by itself. Your program will need some files to run. stdio.h This is the header file and the compiler knows the location of this file.
To use this header file in your program, you must link it through #include the preprocessor.
Why do you need to ad stdio.h file to this program ?
In this program we printf() have used the function which will display the text inside the quotation marks on the screen or console. printf() To use the function you must stdio.h include the header file in your program.
C main() function
In a C program, code execution starts from the main() function. In this case main() it doesn’t matter if the function is not initialized.
The code inside the curly braces {} is main() the body of the function. main() Functions are mandatory in C programs.
int main() {
//function body
}
The above program does nothing. But this is a valid C program.
C function printf()
printf() Functions are library functions that output to the screen. In our program it is on the screen Hello, World! will output.
Remember, you stdio.h need to add a header file to your program to do this.
Notice that there is a semiclon at the end of the print function. A semicolon is used to end a statement.
C return Statement
The return statement terminates the work or the function 0 by returning. main() This statement is not binding. But it is very good to use it in programming.
Essential Rules to Remember
- All C programs main() begin with functions and are mandatory.
- C is case-sensitive. That is, lowercase letters and uppercase latters are used in different meanings.
-
You can use the necessary header files that your program may need. For example:
sqrt() You can use the function to find th square root of a number and pow() you can use the function to find the power. For this you math.h need to add header file to your program.
-
main() A C program terminates whenever a return statement is reached within a C program function. However, main() the return statement in the function is not mandatory.
- Statements in C programs are terminated by a semicolon(;).
Pingback: C Programming Language Keywords -